You need to separate the data and code with appropriate keywords. So, for example, you start off with some declaration of the PIC type and any included files:
; beginning of source
list p=16F684
#include <p16F684.inc>
Next, you can declare variables in a data section (this is just a generic example):
; declaring variables
GENVAR UDATA ; general variables
myvar1 res 1 ; one byte
myvar2 res 2 ; two bytes
Normally, you then start the code section, using the keyword CODE
, and add a goto from the reset vector (where the processor starts executing):
; start of code (reset vector)
RESET CODE 0x0000 ; processor reset vector
goto start
Then, you declare any further CODE sections, for example:
; main program
MAIN CODE
start
; e.g. configure ports
banksel TRISA ; configure PORTA as all o/p
clrf TRISA
; main loop
main_loop
; do stuff
; "
; repeat forever
goto main_loop
; mandatory terminator
END
I think your problem is that you have started writing code without declaring a CODE
section.
EDIT Just fixing your code by adding the CODE
keyword and the initial jump from the reset vector compiles fine:
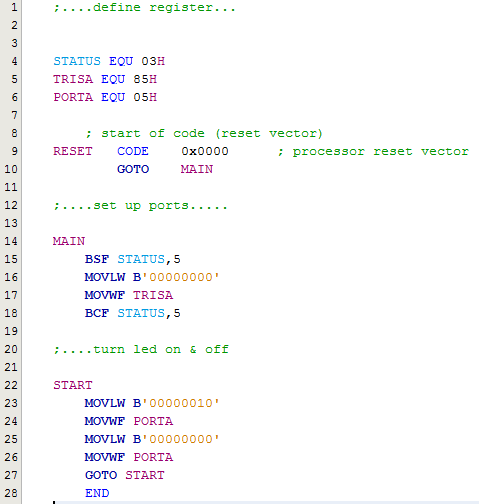
You also need to make sure you respect the necessary indents/initial spaces in code lines so that they are not mistaken for labels. Your first block of code (under MAIN) was not indented, so also caused errors.