What you see on a console window is the result of an automatic echo (which can often be turned off or on), where the console program draws the characters you send on the screen, or the result of receiving characters. This means there are two ways of changing it:
- Have a local program, on the PC, redraw the screen.
- Have the connected device redraw the screen.
There are many ways to do this, but this is how I'd approach a simplistic hobby implementation:
- Set a default line width, like 80, and number of rows, like 24. Turn off automatic echo.
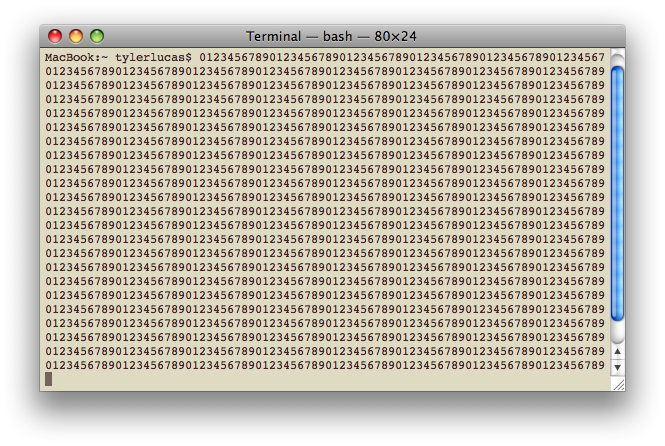
- An 80x24 screen means 1920 characters per 'frame', which means, if the connected device is doing the work, a maximum refresh rate of around
1920/BAUDRATE/11
or about 5Hz at 115200 baud (using 1 start bit, 1 stop, 1 parity, 8 data).
- Map the arrow keys to specific characters or strings. This may already be done behind the scenes. (For OSX Terminal, left:
\033[5D
; right:\033[5C
; up & down undefined by default.)
- Program the connected device to recognize these characters and/or strings, and move the cursor reference accordingly.
- Output over the serial line not by character or character array, but by frame. Specifically, keep a two 1921 (1920+terminator) character-long arrays: one to write to while the other is being transmitted (assuming the use of hardware UART and interrupts).
- Writing to the frame buffer can be accomplished by using a function like this:
uint8_t framewrite(const char *s, uint8_t row, uint8_t col)
... Where *s
is your [terminated] char array, and row
and col
are where you want the first character to appear. (Return uint8_t
is for error catching.)
- You'll probably want functions like
uint8_t frame_clear(void)
, uint8_t frame_shiftrowsdown(void)
, etc.
- To indicate cursor position you can alternate the current character and a black box or the character of your choice (
pblinkchar
), using framewrite(pblinkchar,cursor_row,cursor_col)
.
- The same method can be used to echo characters back to the cursor position.
I'm sure there are much simpler (I think software guys call it "elegant") ways to do this by programming a PC window, and you can probably figure out how by digging through this tutorial, but the above is similar to what I've done in the past and works for simple user interfaces with micros with plenty of spare memory.